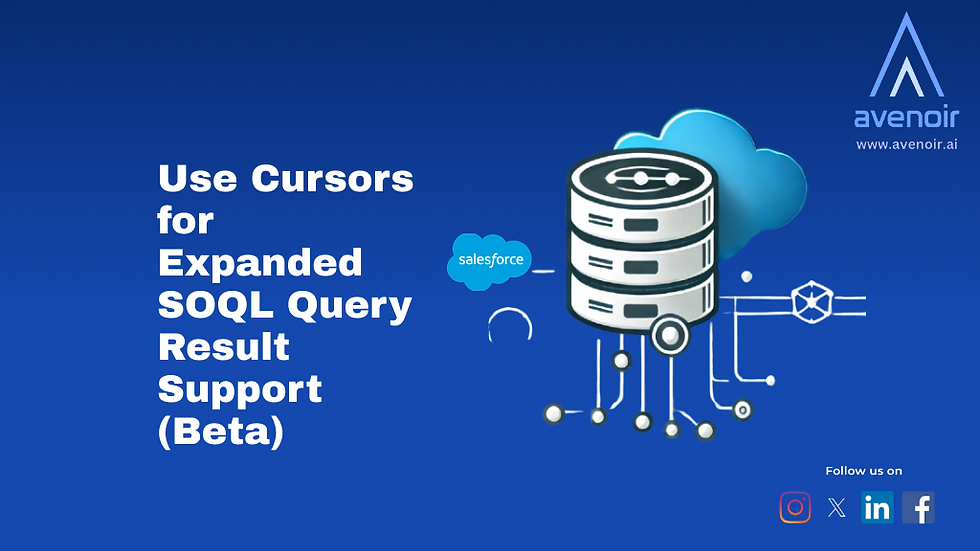
Have you ever struggled with handling large datasets in Salesforce without running into governor limits? Or wondered if there’s a better way to process SOQL query results without relying solely on batch Apex? If so, you’re not alone. Salesforce’s introduction of Apex cursors is here to change the game for developers managing high data volumes. This new feature enables you to process large query results efficiently, one chunk at a time, while maintaining optimal performance.
In this blog, we’ll explore everything you need to know about Apex cursors—how they work, their key features, limitations, and practical examples to help you start using them in your projects.
What are Apex Cursors?
Apex cursors are a powerful feature that lets developers handle large query result sets without loading all the data into memory at once. Instead, you can process the results in smaller, controlled chunks. This is particularly useful in high-volume scenarios where traditional methods like batch Apex might not be the best fit.
Unlike batch Apex, which processes data in fixed batches, cursors give you more flexibility to customize how data is retrieved and processed. They can even integrate seamlessly with queueable Apex jobs, making them ideal for complex workflows.
Key Features of Apex Cursors:
Stateless Operation: Cursors don’t maintain state across calls, allowing better resource handling in multi-transaction environments.
Chunked Processing: Fetch specific numbers of records using offsets and counts, effectively managing governor limits.
Queueable Integration: Easily pair cursors with queueable jobs to process records over multiple executions.
Why Use Cursors?
Handling large datasets in Salesforce often presents challenges like hitting governor limits or inefficient resource utilization. While batch Apex offers a solution, it’s not always the most optimal for scenarios that require dynamic chunk sizes or integration with other processes. Apex cursors are designed to:
Process up to 50 million rows per cursor.
Perform up to 10 fetch calls per transaction.
Handle 100 million rows per day, making them ideal for heavy data operations.
By breaking down the processing of SOQL query results, cursors allow for better control and improved performance compared to traditional approaches.
How Do Cursors Work?
To create an Apex cursor, you can use the Database.getCursor() or Database.getCursorWithBinds() methods.
After executing a SOQL query, you can call the Cursor.fetch(integer position, integer count) method, which takes two parameters: the offset position and the number of records to fetch.
Here’s a breakdown of the key steps in using cursors:
Creating a Cursor: You instantiate a cursor with your SOQL query.
String query = 'SELECT Id, Name FROM Account';
locator = Database.getCursor(query);
Fetching Records: You fetch records using the cursor's fetch method, specifying the position and count.
List<Account> scope = locator.fetch(position, 100);
Processing Records: After fetching, you can perform operations on the records, such as archiving or deletion.
Handling Next Chunks: If more records are needed, you can enqueue the same job to process the next chunk.
Example Implementation:
Let’s look at a practical example of using cursors in a queueable class. The following code demonstrates how to process contact records in chunks:
public class QueryChunkingQueuable implements Queueable {
private Database.Cursor locator;
private Integer position;
public QueryChunkingQueuable() {
String query = 'SELECT Id, Name FROM Account';
// Use the variable to initialize the query locator
locator = Database.getCursor(query);
position = 0;
}
public void execute(QueueableContext ctx) {
List<Account> scope = locator.fetch(position, 200);
position += scope.size();
// Process the fetched records
for(Account acc : scope){
String name = acc.Name;
}
// If there are more records to process, re-enqueue the job
if (position < locator.getNumRecords()) {
System.enqueueJob(this);
}
}
}
In this example, the QueryChunkingQueueable class uses an Apex cursor to fetch contact records in chunks of 200. The execute method processes each chunk and checks if there are more records to fetch. If so, it enqueues itself for the next round of processing.
Considerations and Limitations
While Apex cursors provide significant benefits, there are some important points to keep in mind:
Beta StatusCursors are currently in beta and are subject to Salesforce’s Beta Services Terms.
Exception HandlingBe prepared to handle exceptions like System.FatalCursorException and System.TransientCursorException. The latter can typically be retried.
Governor LimitsCursors share expiration limits with API query cursors, so they must be managed carefully in long-running processes.
Conclusion
Apex cursors represent a powerful tool for developers looking to handle large data sets efficiently in Salesforce. By breaking down the processing of SOQL query results, cursors enhance performance, reduce resource consumption, and provide more flexibility than traditional batch processes. As Salesforce continues to innovate, leveraging these features can lead to more efficient and scalable applications.
If you'd like to see the code and resources used in this project, you can access the repository on GitHub.To access the AVENOIRBLOGS repository, click here. Feel free to explore the code and use it as a reference for your own projects.
Happy Coding! You can leave a comment to help me understand how the blog helped you. If you need further assistance, please contact us. You can click "Reach Us" on the website and share the issue with me.
Reference
https://www.avenoir.ai/post/how-to-use-soql-and-sosl-query-in-salesforce
https://www.avenoir.ai/post/automation-of-data-table-using-fieldset-in-lwc
https://www.avenoir.ai/post/how-to-monitor-setup-methods-using-apextestresult
https://developer.salesforce.com/docs/atlas.en-us.apexcode.meta/apexcode/apex_cursors.htm
Blog Credit:
S. Dash
Salesforce Developer
Avenoir Technologies Pvt. Ltd.
Reach us: team@avenoir.ai
Comments