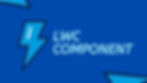
When I joined Avenoir, I was assigned the task to insert multiple records using the LWC component. In this blog, I am going to share how this requirement was achieved.
We will be utilizing lightning-record-edit-form containing adequate lightning-input fields and lightning-layout multiple-rows to handle the addition and deletion of records. In addition, We will be using ShowToastEvent for displaying messages for failed / successful insertion of records, and NavigationMixin for redirecting to the Accounts List page.
OUTPUT:
The output of the code will come out like this:-

Sample insertion of records namely test1,test2 ,test3.


insertMultipleAccounts.html
We are going to use for:each directive to iterate over a list of records for account. As discussed, We are using lightning-record-edit-form component to create form to add or update Salesforce Account records and lightning-input-field components in lightning-record-edit-form component to specify editable fields.
<template>
<lightning-card>
<h3 slot="title">
<lightning-icon icon-name="standard:timesheet" alternative-text="Event"
size="small"></lightning-icon>
{label.PAGE_TITLE}
</h3>
<template for:each={itemList} for:item="item" for:index="index">
<lightning-record-edit-form key={item.id} object-api-name="Account">
<lightning-messages> </lightning-messages>
<lightning-layout multiple-rows>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<lightning-input-field field-name={label.ACCOUNT_NAME} variant="label-stacked"
required>
</lightning-input-field>
</lightning-layout-item>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<lightning-input-field field-name={label.ACCOUNT_PHONE} variant="label-stacked"
required>
</lightning-input-field>
</lightning-layout-item>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<lightning-input-field field-name={label.ACCOUNT_TYPE} variant="label-stacked"
required>
</lightning-input-field>
</lightning-layout-item>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<lightning-input-field field-name={label.ACCOUNT_INDUSTRY} variant="label-stacked"
required>
</lightning-input-field>
</lightning-layout-item>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<lightning-input-field field-name={label.ACCOUNT_NUMBER} variant="label-stacked"
required>
</lightning-input-field>
</lightning-layout-item>
<lightning-layout-item size="12" large-device-size="2" padding="around-small">
<div class="slds-p-top_medium">
<lightning-icon icon-name="action:new" access-key={item.id} id={index}
alternative-text="Add Row" size="small" title={label.ADD_ROW}
onclick={addRow}>
</lightning-icon>
<lightning-icon icon-name="action:delete" access-key={item.id} id={index}
alternative-text="Delete Row" size="small" title={label.DELETE_ROW}
onclick={removeRow}>
</lightning-icon>
</div>
</lightning-layout-item>
</lightning-layout>
</lightning-record-edit-form>
</template>
</br>
<lightning-layout>
<div class="slds-align_absolute-center">
<lightning-button variant="success" onclick={handleSubmit} name={label.SUBMIT_REQUEST}
label="Submit">
</lightning-button>
</div>
</lightning-layout>
</lightning-card>
</template>
Also, see - Display API Responses using LWC Component
insertMultipleAccounts.js
insertMultipleAccounts.js - Here we will write the logic to add and update the list of account records.

addRow() — This method will add value to the array using the push method, resulting in the addition of rows.
addRow() {
++this.keyIndex;
let newItem = [{ id: this.keyIndex }];
this.itemList.push(newItem);
}
removeRow() — This method will filter the array to remove the row. As a result, the row will be no longer be deleted.
removeRow(event) {
if (this.itemList.length >= 2) {
this.itemList = this.itemList.filter(function (element) {
returnparseInt(element.id) !== parseInt(event.target.accessKey);
});
}
}
handleSubmit() — This method validates the input fields before Lightning Data Service to upsert the records. NavigationMixin is being used to navigate to the Account Home page to see the upserted records. ShowToastEvent is being used to send a toast success, error, or warning message on submission.
handleSubmit() {
let isVal = true;
this.template.querySelectorAll('lightning-input-field').forEach(element => {
isVal = isVal && element.reportValidity();
});
if (isVal) {
this.template.querySelectorAll('lightning-record-edit-form').forEach(element => {
element.submit();
});
dispatchSuccessEvent(SUCCESS_TITLE, SUCCESS_MESSAGE);
// Navigate to the Account home page
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Account',
actionName: 'home',
},
});
} else {
dispatchErrorEvent(ERROR_TITLE, ERROR_MESSAGE);
}
}
insertMultipleAccounts.js
The complete javascript code for the requirement is as follows:
import { LightningElement, track } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
import {dispatchSuccessEvent, dispatchErrorEvent} from 'c/dispatchEvent'
import PAGE_TITLE from '@salesforce/label/c.Title_of_the_page';
import ACCOUNT_NAME from '@salesforce/label/c.Show_Account_Name';
import ACCOUNT_ID from '@salesforce/label/c.Show_Account_Id';
import ACCOUNT_TYPE from '@salesforce/label/c.Account_Type';
import ACCOUNT_PHONE from '@salesforce/label/c.Show_Account_Phone';
import ACCOUNT_NUMBER from '@salesforce/label/c.Show_Account_Account_Number';
import ACCOUNT_INDUSTRY from '@salesforce/label/c.Show_Account_Industry';
import SUCCESS_TITLE from '@salesforce/label/c.Success_Title';
import ERROR_TITLE from '@salesforce/label/c.Error_Title';
import SUCCESS_MESSAGE from '@salesforce/label/c.Success_Message';
import ERROR_MESSAGE from '@salesforce/label/c.Error_Message';
import ADD_ROW from '@salesforce/label/c.Add_Row';
import DELETE_ROW from '@salesforce/label/c.Delete_Row';
import SUBMIT_REQUEST from '@salesforce/label/c.Submit_Request';
export default class EditMultipleRecords extends NavigationMixin(LightningElement) {
keyIndex = 0;
label = {
PAGE_TITLE,
ACCOUNT_NAME,
ACCOUNT_ID,
ACCOUNT_TYPE,
ACCOUNT_PHONE,
ACCOUNT_NUMBER,
ACCOUNT_INDUSTRY,
ADD_ROW,
DELETE_ROW,
SUBMIT_REQUEST
}
@track itemList = [
{
id: 0
}
];
addRow() {
++this.keyIndex;
let newItem = [{ id: this.keyIndex }];
this.itemList.push(newItem);
}
removeRow(event) {
if (this.itemList.length >= 2) {
this.itemList = this.itemList.filter(function (element) {
return parseInt(element.id) !== parseInt(event.target.accessKey);
});
}
}
handleSubmit() {
let isVal = true;
this.template.querySelectorAll('lightning-input-field').forEach(element => {
isVal = isVal && element.reportValidity();
});
if (isVal) {
this.template.querySelectorAll('lightning-record-edit-form').forEach(element => {
element.submit();
});
dispatchSuccessEvent(SUCCESS_TITLE, SUCCESS_MESSAGE);
// Navigate to the Account home page
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Account',
actionName: 'home',
},
});
} else {
dispatchErrorEvent(ERROR_TITLE, ERROR_MESSAGE);
}
}
}
insertMultipleAccounts.js-meta
Add the <target> where you want to expose the component under the <targets> tag.
We will be exposing this component to lightning__AppPage, lightning__RecordPage, lightning__HomePage.
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>54.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Happy!! To help you add to your knowledge. You can leave a comment to help me understand how the blog helped you. If you need further assistance, please leave a comment or contact us. You can click on "Reach us" on the website and share the issue with me.
References:
lightning-navigation - documentation - Salesforce Lightning Component Library
Display Toast Notifications - Salesforce Lightning Component Library
lightning-record-edit-form - documentation - Salesforce Lightning Component Library
lightning-input-field - documentation - Salesforce Lightning Component Library
Navigate to Different Page Types - Salesforce Lightning Component Library
Blog Credit:
Z. G. Mir
Salesforce Developer
Avenoir Technologies Pvt. Ltd.
Reach us: team@avenoir.ai