Hey Learners! Sorting in Salesforce Apex is a common requirement when working with collections of data, such as Lists or Sets. While Salesforce provides default sorting methods, there are scenarios where custom sorting based on specific criteria or locale is necessary. You can use the Comparator Interface and the Collator Class to achieve this. In this blog post, we'll explore how to use these tools for sorting in Salesforce Apex.
Understanding the Comparator Interface
The Comparator interface in Apex is a pathway to defining custom sorting logic. It's beneficial when the default sorting methods don’t meet your specific requirements. By implementing this interface, you can dictate exactly how objects should be compared and sorted.
Comparator Interface: Allows the definition of a custom compare method to sort objects in a user-defined order.
The Power of the Collator Class
When dealing with international data, sorting can get complicated. This is where the Collator class comes into play. It's designed for complex sorting scenarios, particularly for sorting strings in a way that respects locale-specific rules.
Collator Class: Facilitates locale-sensitive string comparison, crucial for applications that operate on a global scale.
The List class now supports the new Comparator interface, so you can implement different sort orders in your code by using List.sort() with a Comparator parameter. To perform locale-sensitive comparisons and sorting, use the getInstance method in the new Collator class. Because locale-sensitive sorting can produce different results depending on the user running the code, avoid using it in triggers or in code that expects a particular sort order.
Let's take an example of a student class that has few properties like student name, phone, and Id.
public with sharing class StudentRecord {
public String studentName;
public String Phone;
//Constructor
public StudentRecord(String studentName, String Phone) {
this.studentName = studentName;
this.Phone = Phone;
}
}
Now using the “Comparator” interface, we can write multiple classes to sort based on different properties of the StudentRecord class.
public with sharing class CompareByStudentName implements Comparator<StudentRecord> {
public Integer compare(StudentRecord studentOne, StudentRecord studentTwo) {
Integer returnValue = -1;
if(String.isNotBlank(studentOne.studentName)) {
returnValue = String.isBlank(studentTwo.studentName) ? 1 :
studentOne.studentName.compareTo(studentTwo.studentName
);
}
return returnValue;
}
}
Now, let's test the above code using some sample data. I have the following anonymous window code to test this out.
List<StudentRecord> students = new List<StudentRecord>{
new StudentRecord('John Doe','234564'), new
StudentRecord('Ayne Smith', '876543')
};
System.debug('before sorting');
for(StudentRecord student : students) {
System.debug(student.studentName + ' - ' + student.Phone);
}
CompareByStudentName compareByStudentName = new CompareByStudentName();
// Sort
students.sort(compareByStudentName);
System.debug('after Sorting');
for(StudentRecord student : students) {
System.debug(student.studentName + ' - ' + student.Phone);
}
See the results
Conclusion
The Comparator interface and Collator class in Salesforce Apex allow for custom sorting of data collections, enabling developers to define specific sorting logic beyond default methods. The Comparator interface facilitates user-defined sorting orders, while the Collator class provides locale-sensitive string comparison, essential for applications with global data. These tools enhance data handling capabilities in Apex, offering tailored sorting solutions to meet complex requirements.
References
Blog Credit:
S. Yadav
Salesforce Developer
Avenoir Technologies Pvt. Ltd.
Reach us: team@avenoir.ai
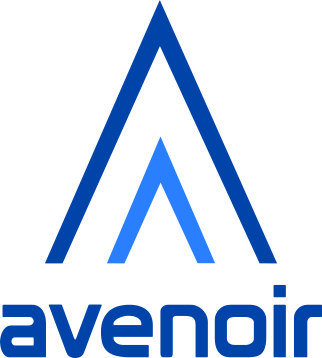
Are you in need of Salesforce Developers?
Reach Us Now!
Comments